This is a quick Python cheat sheet with Examples. It contain two parts:
- Python basics
- This is just a basic overview
- Advanced topics
- many more features and concepts in Python
Python basics cheat sheet
Python data types
data |
example |
explanation |
Integer |
7 |
whole numbers |
Float |
4.2 |
floating-point numbers |
String |
"Hello" |
sequence of characters |
Boolean |
True; False |
booleans |
List |
[ 1, 2, 3 ] |
ordered collection of items |
Tuple |
( 1, 2, 3 ) |
immutable collection of items |
Dictionary |
{ "a": 1, "b": 2 } |
unordered collection of pairs |
Set |
{ "a", "b", "c"} |
unordered collection of unique items |
Basic Python data types
- int (integers) - whole numbers
- float (floating-point numbers) - numbers with a decimal point
- str (strings) - sequences of Unicode characters
- bool (booleans)
Python Collections
- list - an ordered collection of items
- tuple - an ordered, immutable collection of items
- dict (dictionaries) - an unordered collection of key-value pairs
- e.g. {'name': 'John', 'age': 30}
- set - an unordered collection of unique items
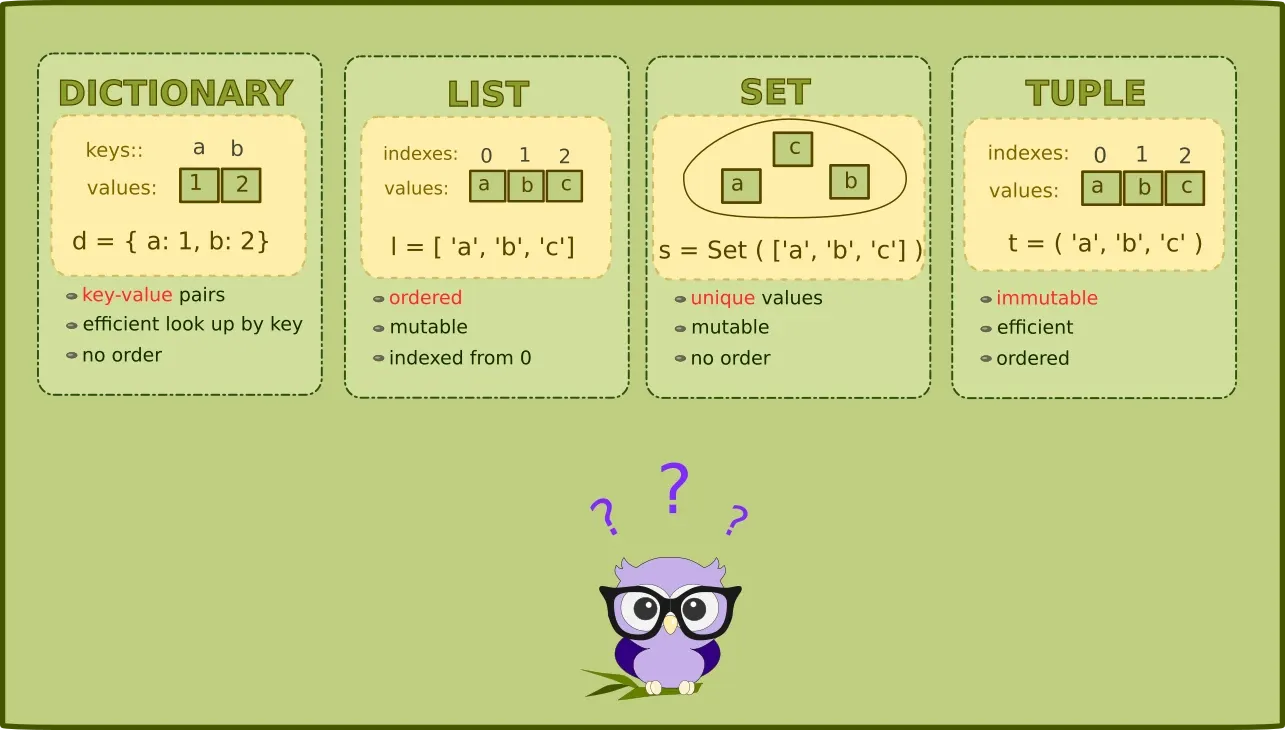
Type casting
function |
explanation |
int(expr) |
Converts expression to integer |
float(expr) |
Converts expression to float |
str(expr) |
Converts expression to string |
chr(num) |
ASCII char num |
Python Control structures
- if-elif-else - used to make decisions
- for - used to iterate over a collection or a range of numbers
- while - used to repeat a block of code until a condition is met
if-elif-else example
x = 4
y = 7
if x > y:
print("x > y")
elif x < y:
print("x < y")
else:
print("x = y")
for loop
pets = ["cat", "dog", "mouse"]
for pet in pets:
print(pet)
while example
i = 1
while i < 3:
print(i)
i += 1
Functions in Python
- def - used to define a function
- return - used to return a value from a function
def some_function():
print("Hello from a function")
def another_function():
return 1
some_function()
print(another_function())
Classes
- class - used to define a class
- self - used to refer to the current instance of a class
class Student:
def __init__(self, name, age):
self.name = name
self.age = age
s1 = Student("Dan", 17)
s2 = Student("Sam", 15)
print(s1.name)
print(s1.age)
Modules
- import - used to import a module
import math
from math import pi
from math import pi as PI
from . import package
Other
- None - represents a null value
- lambda - used to create small anonymous functions
- with - used to wrap the execution of a block of code with methods defined by a context manager
lambda example
x = lambda a : a + 1
print(x(3))
x = lambda a, b : a + b
print(x(3, 4))
x = lambda a, b, c : a * b * c
print(x(1, 2, 3))
with example
with open('file_path', 'w') as f:
f.write('Hello world')
Exception Handling
f = open("demofile.txt")
try:
f.write("Some text")
except:
print("Error on file write")
finally:
print("Always executed")
f.close()
Python advanced cheat sheet
Arithmetic Operators
operator |
name |
x + y |
add |
x * y |
multiply |
x % y |
modulus |
x - y |
subtract |
x / y |
divide |
x ** y |
power |
Comparison Operators
operator |
name |
x < y |
Less |
x > y |
Greater |
x == y |
Equal |
x <= y |
Less or eq |
x >= y |
Greater or eq |
x != y |
Not equal |
Boolean Operators
operator |
name |
not x |
Not |
x and y |
And |
x or y |
Or |
More Python operations
operation |
explanation |
len(s) |
length of s |
s[i] |
i-th item in s (0-based) |
s[start : end] |
slice of s from start (included) to end (excluded) |
x in s |
True if x is contained in s |
x not in s |
True if x is not contained in s |
s + t |
the concatenation of s with t |
s * n |
n copies of s concatenated |
sorted(s) |
return a sorted copy of s |
s.index(item) |
return position in s of item |
List operations
function |
explanation |
del lst[i] |
Deletes i-th item from list |
lst.append(e) |
Appends element to list |
lst.insert(i, e) |
Inserts e before i-th item in l-st |
lst.sort() |
Sorts list |
Dictionary operations
function |
explanation |
len(d) |
Number of items in dict |
del d[key] |
Removes key from dict |
key in d |
True if d contains dict |
d.keys() |
Returns keys from dict |
String Operations
String manipulation
example |
explanation |
"Hello"; 'world' |
Strings examples |
s1+s2 |
concatenate |
s1*3 |
multiply |
s1 == s2 (or != or > or <) |
compare ('if' or 'if not') |
'wo' in 'world' |
check ('if or 'if not') |
s1[0] or s1[-1] or s1[x] |
position |
s1[start:end:step] |
start - inc, end - exclusive |
s1[:3] or s1[1:] |
slicing |
s1[::-1] |
invert string |
Most used string operations
function |
explanation |
s.lower() |
return lowercase copy of s |
s.replace('old', 'new') |
replace 'old' with 'new' |
s.split( 'sep' ) |
list of substrings split by 'sep' |
s.strip() |
return s without whitespaces |
s.upper() |
return uppercase copy of s |
f-string
year = 2016
event = 'Referendum'
f'Results of the {year} {event}'
'Results of the 2016 Referendum'
txt = "This is {0}, I'm {1}".format("Dan",17)
'This is Dan, I'm 17'
numbers
"The price is ${0:,.2f}".format(100.3373)
'The price is $100.34'
dates
from datetime import datetime
now = datetime.now()
'{:%Y-%m-%d %H:%M:%S}'.format(now)
'2023-01-05 11:03:55'
Slicing
Indexes and Slices of a = [0, 1, 2, 3, 4, 5]
. Python slicing of string and lists works as follows:
string[start:end:step]
list[start:end:step]
- start is included, end - excluded
- Positive number - from left to right
- Negative number - from right to left
operation |
result |
explanation |
len(a) |
6 |
length |
a[0] |
0 |
first item |
a[5] |
5 |
last item by index |
a[-1] |
5 |
last item by -1 / first from right |
a[-2] |
4 |
second from right to left |
string[2:5] |
[2,3,4] |
from start to end |
a[1:] |
[1,2,3,4,5] |
all items up to 5th |
a[:5] |
[0,1,2,3,4] |
all items up to -2 |
a[:-2] |
[0,1,2,3] |
from start to end - from 2nd to 3rd |
a[1:3] |
[1,2] |
from 2nd to the last |
a[1:-1] |
[1,2,3,4] |
from start to end |
a[::-1] |
[5,4,3,2,1,0] |
reverse list order |
a[::-2] |
[5,3,1] |
step by 2 reverse |
b=a[:] |
[0, 1, 2, 3, 4, 5] |
shallow copy of list |
from datetime import datetime
time = datetime.now()
print("no formatting:", time)
print("formatting:", time.strftime("%X"))
directive |
result |
example |
%a |
Abbreviated weekday (Sun) |
Thu |
%A |
Weekday (Sunday) |
Thursday |
%b |
Abbreviated month name (Jan) |
Jan |
%B |
Month name (January) |
january |
%c |
Date and time |
Thu Jan 5 14:50:48 2023 |
%d |
Day (leading zeros) (01 to 31) |
05 |
%H |
24 hour (leading zeros) (00 to 23) |
14 |
%I |
12 hour (leading zeros) (01 to 12) |
02 |
%j |
Day of year (001 to 366) |
005 |
%m |
Month (01 to 12) |
01 |
%M |
Minute (00 to 59) |
53 |
%p |
AM or PM |
PM |
%S |
Second (00 to 29) |
48 |
%U |
Week number (00 to 53) |
01 |
%w |
Weekday (0 - Sun to 6 - Sat) |
4 |
%W |
Week number (00 to 53) |
01 |
%x |
Date |
01/05/23 |
%X |
Time |
14:56:48 |
%y |
Year without century (00 to 99) |
23 |
%Y |
Year (2008) |
2023 |
%Z |
Time zone (GMT) |
GMT |
%% |
A literal %" character (%) |
% |
Python sys.argv
python sample.py Hello Python
- sys.argv[0] == 'sample.py'
- sys.argv[1] == 'Hello'
- sys.argv[2] == 'Python'
Note: sys.argv[0]
is always the filename/script executed
Python environment setup
function |
explanation |
sys.argv |
List of command line arguments (argv[0] is executable) |
os.environ |
Dictionary of environment variables |
os.curdir |
String with path of current directory |
import os
os.environ
OS variables
directive |
result |
example |
curdir |
Current dir string |
. |
defpath |
Default search path |
/bin:/usr/bin |
extsep |
Extension separator |
. |
linesep |
Line separator |
\n |
name |
Name of OS |
posix |
pardir |
Parent dir string |
.. |
pathsep |
Patch separator |
: |
sep |
Path separator |
/ |
List methods
- append(item)
- count(item)
- extend(list)
- index(item)
- insert(position, item)
- pop(position)
- remove(item)
- reverse()
- sort()
- insert(position, item)
More examples and comparison tuples, dict and set here: Python: Compare List vs Tuple vs Dictionary vs Set